Dynamic Memory allocation in C
First let us look into how the memory is organized. The memory is mainly divided into stack, heap and permanent storage area. The Stack is used to store local variables. Global variables, program instructions and static variables are stored in the permanent storage area. Our main concern is heap which is free memory available, allocated dynamically using some standard library functions.
In C, the two key dynamic memory functions are malloc() and free().The prototype for malloc looks like void *malloc(size_t size)
and for free it looks like void free(void *pointer)
Here is an example to illustrate the use of these functions:
The static way of defining an array
int a[100];
a[10] = 25;
The dynamic way of defining an array
int *p;
p = malloc(100 * sizeof(int));
*(pointer+10) = 25;
When the array is no longer needed, it is deallocated using free function
free(p);
p = NULL;
The assigning of NULL to pointer 'p' is optional, but it is done in order to avoid dangling pointer problem
For more understanding refer Dynamic memory allocation in c.
Dynamic Memory allocation in C++
C++ provides two operators new and delete for dynamic memory allocation.
The following syntax shows the use of new operator in various ways
variable = new typename;
variable = new type(initializer); //includes initialization
array = new type [size]; //array of objects
The memory is de-allocated using delete operator as shown:
delete variable;
delete[] array;
Here is an example to create a dynamic 1D array:
int *p;
pointer = new int[100];
pointer[10] = 25;
The pointer p is deallocated using delete:
delete[] p;
p = NULL;
C++ provides another option for dynamic memory allocation using the Standard Template Library.
Dynamic Memory allocation of 2D array in C++
Allocating memory for 2D array is not straight forward like normal 1D array. A dynamic 2D array is an array of pointers to arrays.
The steps used to create a dynamic 2D array are:
int** a = new int*[row_size];
for(int i = 0; i < row_size; ++i){
a[i] = new int[column_size];
}
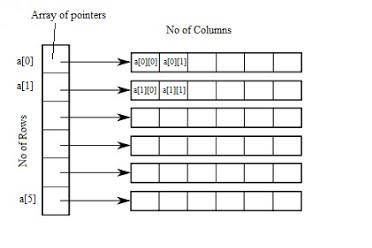
First step creates 1D dynamic array(which is array of pointers) and in second step creates the column of the array. Figure 1 gives a clear idea.
Deallocating dynamic 2D array:
for(int i = 0; i < row_size; ++i) {
delete [] a[i];
}
delete [] a;
Here is an example for creating and deleting dynamic 2D array with 4 rows and 5 columns (i.e a[4][5]):
int** a = new int*[4];
for(int i = 0; i < 4; ++i){
ary[i] = new int[5];
}
for(int i = 0; i < 4; ++i) {
delete [] a[i];
}
delete [] a;
This article is taken from my old blog.