TensorFlow is a multipurpose Opensource software library for numerical computation using data flow graphs. It is originally developed by Google Brain Team to conduct deep neural networks research. TensorFlow is written in Python, C++ and CUDA. It provides stable Python API,C APIs and without backwards compatability gaurantee C++, Go, Java, JavaScript and Swift. TensorFlow is applied in a wide variety of domains as well.The following figure shows how SketchRNN built using TensorFlow will complete your sketch in multiple ways.
Source: magenta.tensorflow.org
In this tutorial I will cover the very basics of TensorFlow. Deep learning will be covered in a different post. I will be using TensorFlow's Python API to explain.
1. Installing TensorFlow
TensorFlow is a library and it can be installed like any other library using pip
command.
- Install python 3.6 and above. Download from here.
- Install Tensorflow
pip3 install --upgrade tensorflow
1.1 Importing TensorFlow
After installing, you can run the import
statement to use TensorFlow library.
2. Tensors
Let's us first understand what are tensors and the reference that we can take to get going.
A scalar is a physical quantity that it represented by a dimensional number at a particular point in space and time. Examples are hydrostatic pressure and temperature.
A vector is a bookkeeping tool to keep track of two pieces of information (typically magnitude and direction) for a physical quantity. Examples are position, force and velocity.
What happens when we need to keep track of three pieces of information
for a given physical quantity?
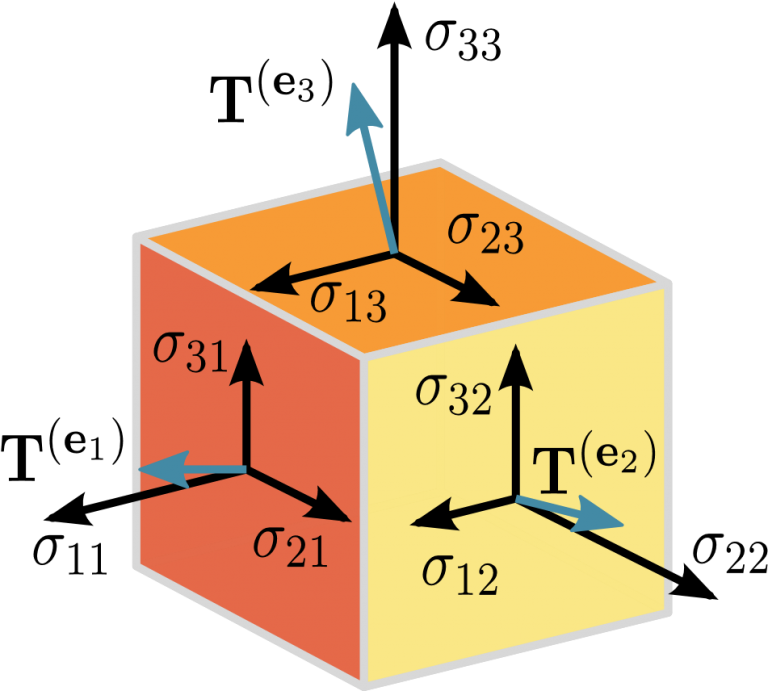
A Tensor has the following properties:
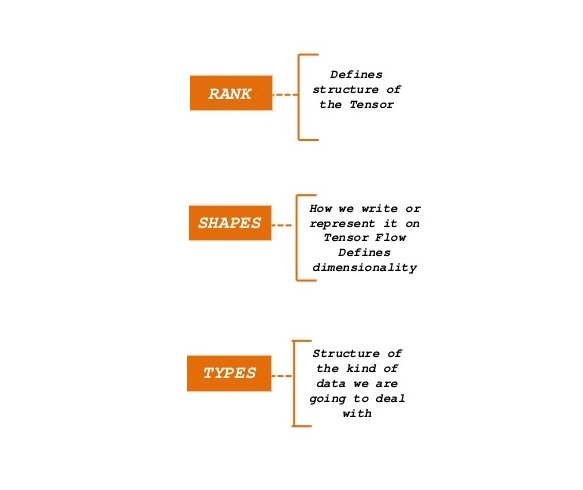
Source: slideshare.net
The rank of a tensor is its number of dimensions.The shape of a tensor is the number of elements in each dimension
The following image gives a better intuition about Tensors.
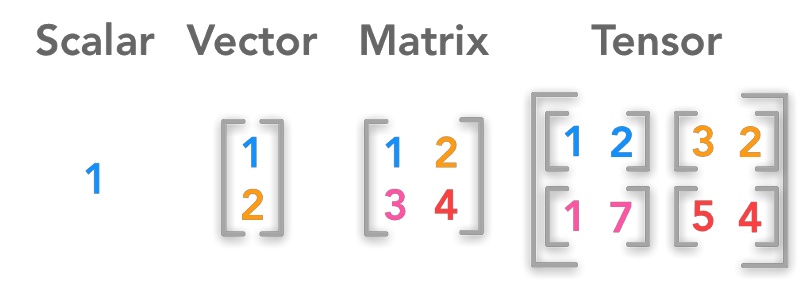
Source: google.com
In simple terms Tensors are called as multidimenional arrays.
3. Computational graph
TensorFlow internally represent the operations and performs its computation using a data flow graph (or computational graph). In other words Tensorflow approaches series of computations as a flow of data through a graph with nodes being computation units and edges being flow of Tensors.
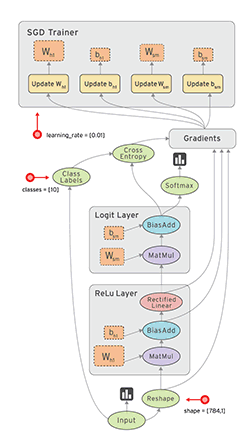
Source: tensorflow.org
Nodes are the operations such as addition or subtraction and edges are the tensors. It consists of two phases
Build the graph
: Here you define the Variables, Constants and Placeholders, and their relations.(define the mathematical operations on them)Execute the graph
: Till now, all the variables and the computations applied. They are computed in this phase
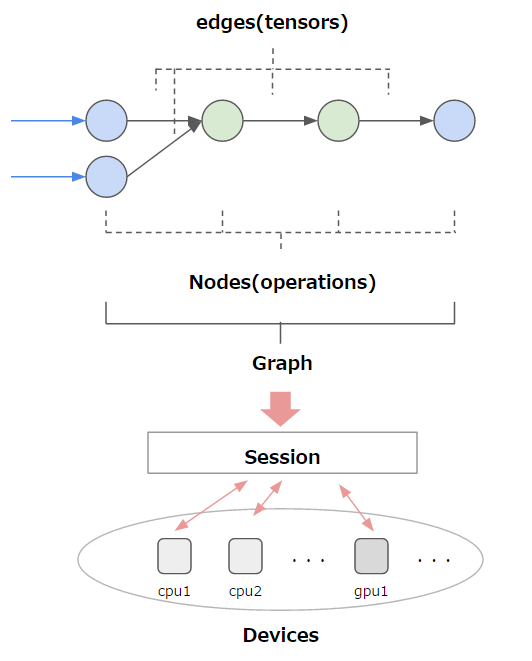
Source: google.com
3.1. Build the graph
3.1.1. Variables
3.1.1. Variables
Let's see the official documentation definition
> A variable maintains state in the graph across calls to run(). You add a variable to the graph by constructing an instance of the class Variable.The Variable() constructor requires an initial value for the variable, which can be a Tensor of any type and shape. The initial value defines the type and shape of the variable. After construction, the type and shape of the variable are fixed. The value can be changed using one of the assign methods.Variables can be created by tf.Variable().
tensorflow_var = tf.Variable(value, name="variable_name",dtype)
Most of the time you will want to create these variables as tensors of zeros, ones or random values:
- tf.zeros() #creates a matrix full of zeros
- tf.ones() #creates a matrix full of ones
- tf.random_normal() #a matrix with random uniform values between an interval
- tf.random_uniform() #random normally distributed numbers
- tf.truncated_normal() 3same as random normal but doesn’t include any numbers more than 2 standard deviations.
3.1.2. Placeholders
Placeholders are nodes whose values are fed in at execution time. In case of supervised learning the input-output pairs are fed in at execution time for training. In other words placeholder lets you inject external data to the computation graph.While declaring placeholders we only assign datatype and shape.
tensorflow_placeholder = tf.placeholder(dtype,shape=None,name=None)
3.1.3. Model
Model is a mathematical function that maps the inputs to outputs using the model variables. For our example we will use
a very simple linear model
In the above line we defined our linear model in which we have defined two computation nodes matmul
and add
.
3.1.4. Loss Measure
In machine learning, error is calculated as the difference between the actual output and the predicted output. The function that is used to compute this error is called loss function. Different loss functions will give different errors. The performance of the model depends on the loss function. One of the most widely used is mean square error, where loss function is calculated as the square of difference between actual value and predicted value which is divided by total samples.
3.1.5. Optimization Method
Optimization Algorithms are used to update weights and biases i.e. the internal parameters of a model to reduce the error. We are going to use Gradient Descent optimizer to optimize our model parameters. We create our optimizer object tf.train.AdamOptimizer
and add an optimization operation on the graph by calling minimize method on the optimizer object. Please see my post on Gradient Descent Algorithm to know about how an optimization algorithm works.
minimize(cost) does two things:
- Computes the gradient of our argument (loss node) with respect to to all the variables (weights and biases).
- Applies gradient updates to all those variables.
3.2. Run the graph
We have constructed the necessary componenets of the computation graph. The next step is to execute it.
3.2.1 Session
A Session object encapsulates the environment in which operation objects are executed, and Tensor objects are evaluated. To execute the graph, we must initialize a Session object, and call its run method.
Create Session object
There are two ways to create the session object as shown below.
Initialize the variables
We need to initialize the variables as we only assign real values to the variables once we are in a runtime i.e. after a session has been created.
Run the method of Session object
The run method of Session object evaluates the output of a node of the graph
4. More Information
4.1 DataTypes
4.1 DataTypes
Tensors have a data type in addition to shape and dimension. The following table shows the various datatypes available.
4.2 Constants
Constant value cannot be changed once create. When it is sure that value will not change, tf.consant is used. It's initialization should be with a value, not with operation.
5. Stitching
Before concluding let's predict the price of the apple stock on a given day. We will use all the code snippets and use them to complete the stock prediction script.
I hope you liked this tutorial. You can find the complete code on my github Linear Regression using TensorFlow. I have used the following references and sometimes used the same explanation. Do check the following resources for more understanding.
- Understanding Tensors and Graphs to get you started in Deep Learning
- An Introduction to Tensors for Students of Physics and Engineering
- What’s the difference between a matrix and a tensor?
- An Introduction to TensorFlow by Liu Songxiang
- TensorFlow in a Nutshell — Part One: Basics by Camron Godbout
- Loss Functions and Optimization Algorithms. Demystified. by Apoorva Agrawal
If you have any question or feedback, please do reach out to me by commenting below.