In the previous post, we saw the basic commands to create a docker image. In this post we will look at docker remote api's.
Understanding Docker Architecture
Before diving into the docker remote api's, visualizing docker architecture will give better intution. Here's a peek at how Docker works under the hood.
Let's learn about the layers starting from the core
- Docker Daemon : is a service that runs on the host operating system. It currently runs on Linux because it depends on a number of Linux kernel features
- REST API : tools can talk to Docker daemon through the REST API exposed by the docker
- Docker CLI : It is a command line tool that can talk to docker daemon(This internally calls REST API exposed by docker daemon). Docker CLI comes along with docker daemon when docker is installed
The Docker architecture is anologous to client-server architecture. The daemon is the server and the CLI is one of many clients.
Now let's look at each component of a docker ecosystem :
- Client : This is where we run the docker commands. It can be CLI or REST API. We generally install CLI on our operating system to run the commands
- Docker host : This is typically referred to as the server running the Docker daemon. The client and daemon need not to be on the same machine
- Registry : Docker registry is a storage and content delivery system holding Docker images available in different tagged version. docker push and pull commands are used to interact with registry. We can create our own local registry if we wish to maintain. We can will explore this in the later posts
Enabling Docker Remote API
The above explanation gives better intution about how Docker Remote API works. In order to enable Docker remote api on your machine (I use Ubuntu) follow the steps mentioned below
- The first step is to check the running docker services. In order to do so use the following command.
If there is a service withps -ef | grep docker
/usr/bin/dockerd -H=fd:// -H=tcp://0.0.0.0:2375
then the next steps can be skipped. Generally for the first time it has to be configured and the output looks like - Then navigate to /lib/systemd/system in the terminal and edit docker.service file vi /lib/systemd/system/docker.service
vi /lib/systemd/system/docker.service
- Find the line which starts with ExecStart and adds -H=tcp://0.0.0.0:2375 to make it look like ExecStart=/usr/bin/dockerd -H=fd:// -H=tcp://0.0.0.0:2375
- Reload the docker daemon

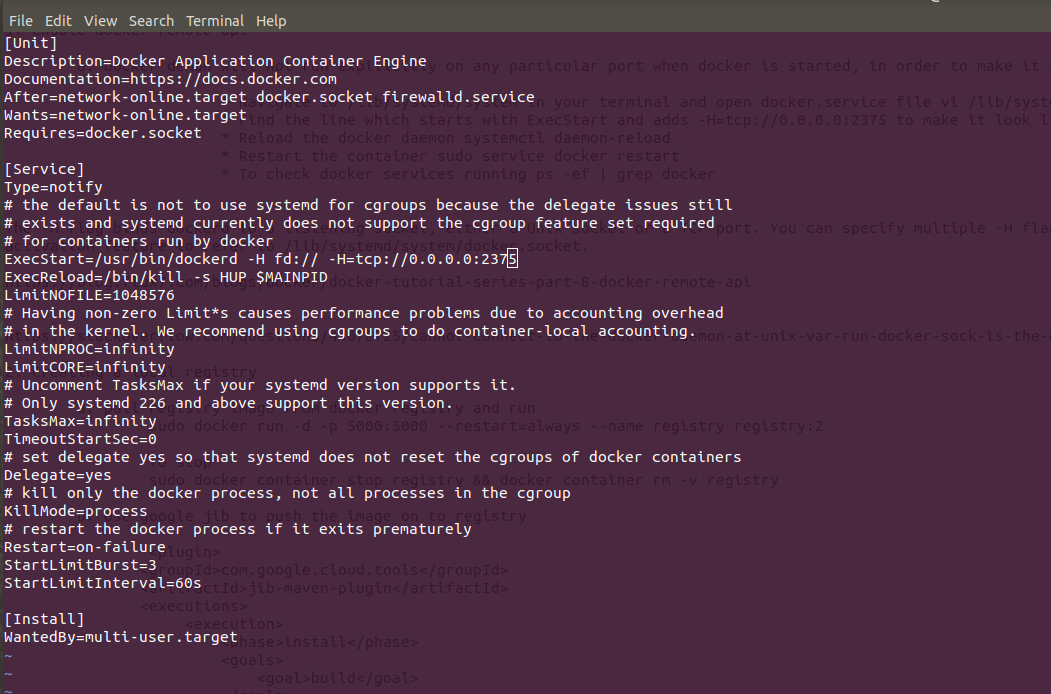
systemctl daemon-reload
sudo service docker restart
ps -ef | grep docker

How to use Docker Remote API
Now that we enabled Docker remote api, it's time to dirty our hands with the api's. Here are few
1. List of images
In the first example, I will use three different ways to fetch the list of images available on the docker machine this helps to understand the analogy between different ways.
- Using cmd :
docker images
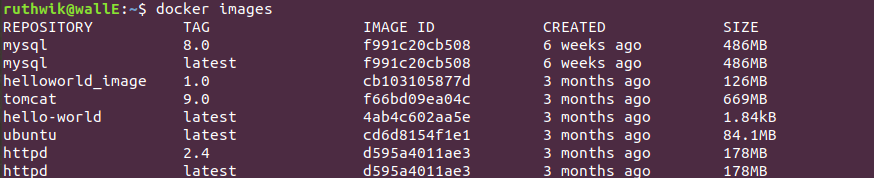
curl http://localhost:2375/images/json


2. List of containers
This is to get list of containers created on the docker machine.
curl http://localhost:2375/containers/json?all=1
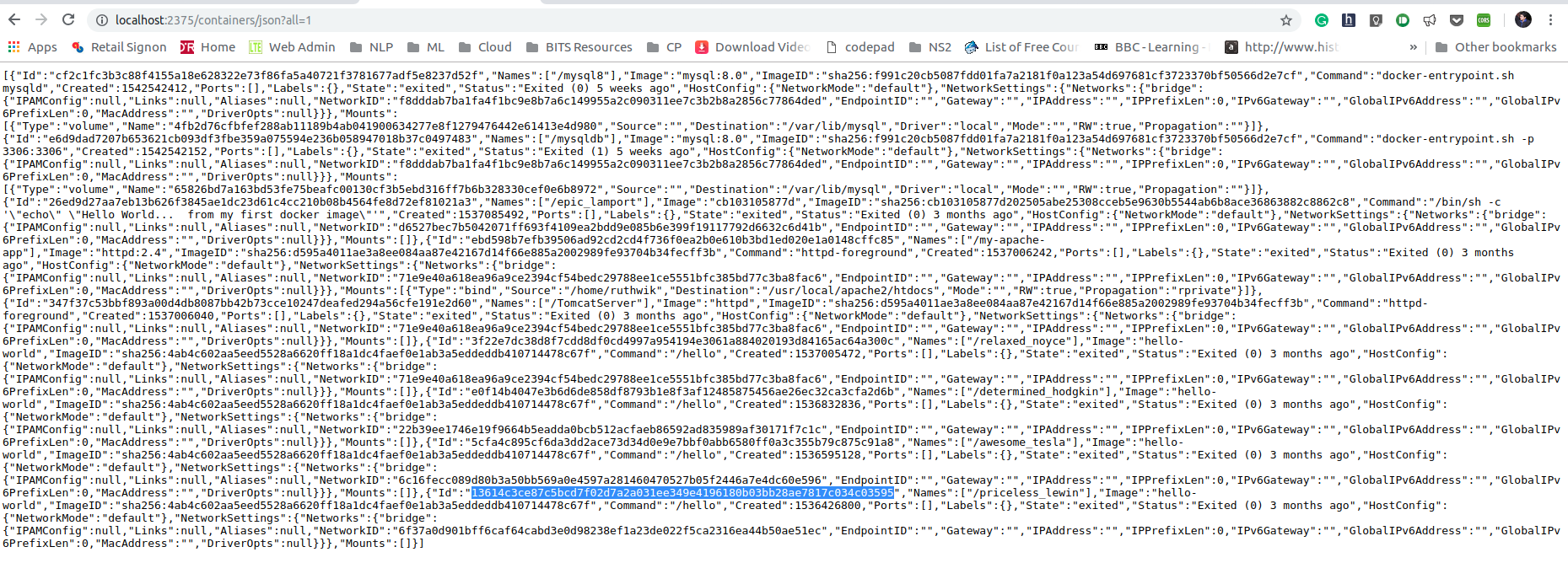
3. Pull an image
This is
curl -XPOST http://localhost:2375/images/create?fromImage=tomcat:9.0
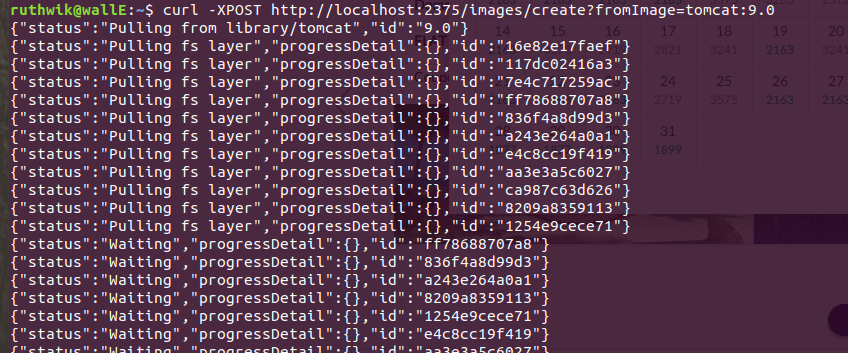
4. Create a container
When running a container via the command line you would use the docker ‘run’ command. This is a composite command, consisting of the commands ‘create’ and ‘start’. The remote API does not support the ‘run’ command. You have to execute ‘create’ and ‘start’ separately.
curl -X POST -H "Content-Type: application/json" http://localhost:2375/containers/create -d '{
> "Image":"tomcat:9.0",
> "PortBindings": { "8080/tcp": [{ "HostPort": "9090" }] }
> }'
This will give an id as output which is used to uniquely identify the container. We use this to start, stop and delete..The port bindings will map the image internal port to external port. Here tomcat's 8080 port is mapped to 9090 port. Now the tomcat is accessable on the 9090 port of the machine.

5. Start a container
Here we give the unique id in the path to start the container
curl -XPOST http://localhost:2375/containers/0be90b6cfec41e89270bab7280b9691773c949f6130f8aaba20dbf2aec533dcf/start

6. Stop a container
Stop the container using the container id.
curl -XPOST http://localhost:2375/containers/0be90b6cfec41e89270bab7280b9691773c949f6130f8aaba20dbf2aec533dcf/stop
7. Kill a container
curl -XPOST http://localhost:2375/containers/0be90b6cfec41e89270bab7280b9691773c949f6130f8aaba20dbf2aec533dcf/kill
I have used the following references and sometimes used the same explanation. Do check the following resources for more understanding.
- How to build an Image using Docker API?
- Offical Docker documentation
- Using the Docker REST API to run a container with parameters
- Docker Tutorial Series, Part 8: Docker Remote API
- Cannot connect to the Docker daemon at unix:/var/run/docker.sock. Is the docker daemon running?
If you have any question or feedback, please do reach out to me by commenting below.